Code Optimization: Efficient Programming
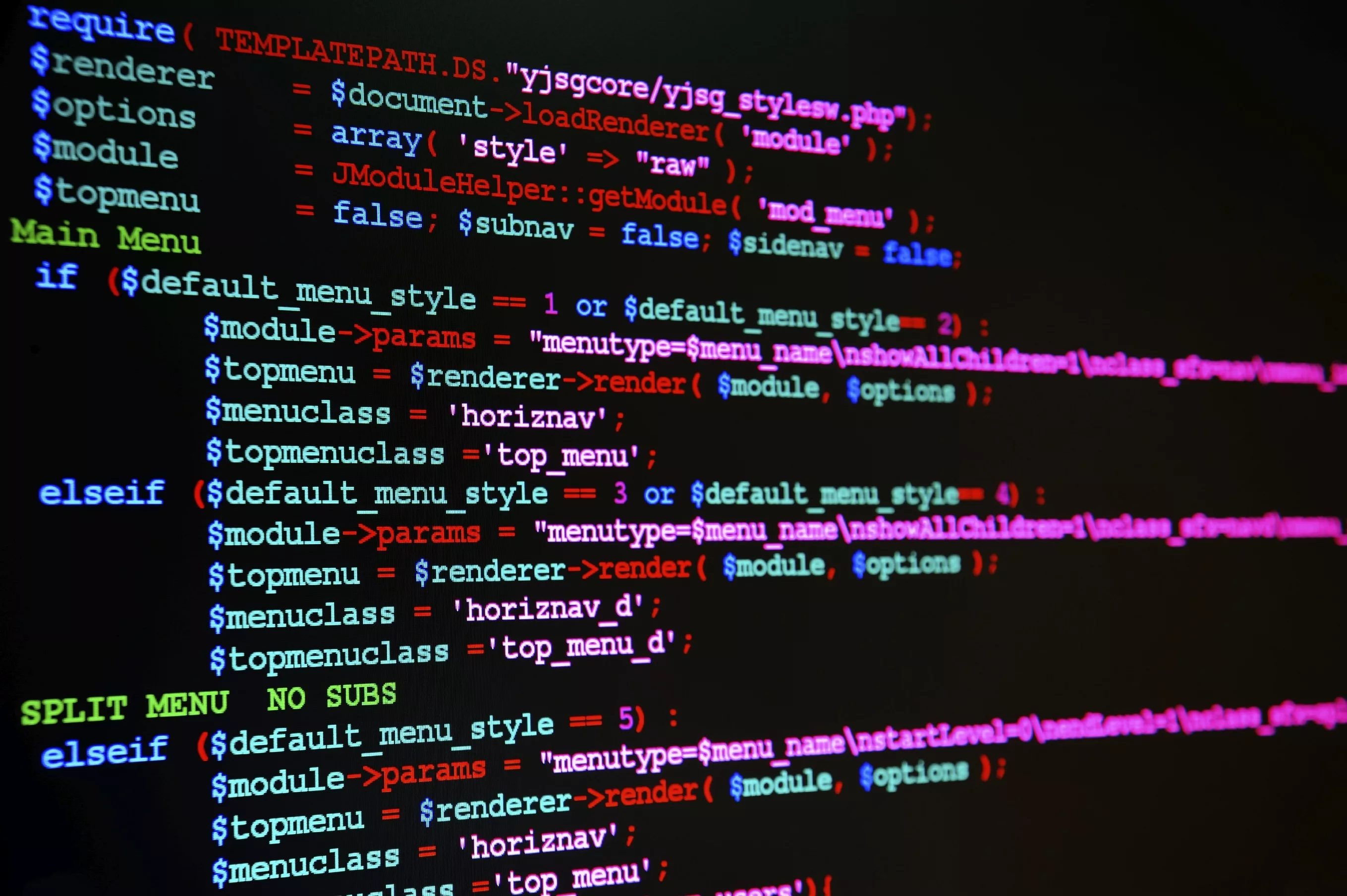
In today's software development landscape, the importance of writing efficient and fast code cannot be overstated. As projects grow in size and complexity, the demand for performance and scalability becomes paramount. Code optimization plays a crucial role in ensuring that applications run smoothly, utilize resources effectively, and provide an excellent user experience. In this article, we will explore key strategies for code optimization, offering practical tips to enhance the performance of large projects.
Understanding Code Optimization
Code optimization refers to the process of modifying code to make it run faster, consume fewer resources, or improve its overall efficiency. It can involve various techniques, including algorithm improvements, data structure optimizations, and code refactoring. While it's essential to focus on writing clean and maintainable code, considering optimization from the start can save time and resources in the long run.
Key Strategies for Code Optimization
1. Choose the Right Algorithms and Data Structures
The choice of algorithms and data structures is fundamental to code performance. A well-optimized algorithm can drastically reduce execution time, while inefficient algorithms can lead to significant slowdowns. Before implementing any functionality, analyze the problem at hand and select the most appropriate algorithm.
For example, if you need to search for elements in a large dataset, consider using a hash table or a binary search tree instead of a linear search. Similarly, understanding the time and space complexities of different data structures can help you make informed decisions that impact overall efficiency.
2. Minimize I/O Operations
Input/output (I/O) operations can be a significant bottleneck in many applications, especially those that handle large volumes of data. Minimizing I/O operations can lead to substantial performance improvements. Here are some tips:
- Batch Processing: Instead of reading or writing data one piece at a time, consider processing data in batches. This reduces the number of I/O operations and can significantly speed up performance.
- Use Caching: Implement caching mechanisms to store frequently accessed data in memory, reducing the need for repeated I/O operations. This can be particularly effective for web applications that query databases frequently.
3. Optimize Loops and Iterations
Loops are a common source of inefficiencies in code. Here are several strategies to optimize loops:
- Avoid Unnecessary Calculations: Move calculations that do not change within the loop outside of it. For instance, if you’re calculating a constant value, compute it once before the loop starts.
- Use Efficient Iteration Constructs: In languages that support it, consider using built-in functions or constructs that are optimized for performance, such as list comprehensions in Python.
- Limit the Loop’s Scope: If possible, minimize the size of the dataset you are iterating over. Filtering data before it enters a loop can lead to faster execution.
4. Reduce Function Call Overhead
Function calls come with overhead costs, especially in performance-critical sections of code. To optimize:
- Inline Functions: Where appropriate, consider inlining small functions to eliminate the overhead of function calls. This is particularly useful for simple getter and setter methods.
- Use Tail Recursion: In recursive functions, if the last action is a recursive call, the compiler can optimize the call stack, effectively converting it into a loop. This can save memory and improve performance.
5. Profiling and Benchmarking
Optimization without measurement is akin to shooting in the dark. Profiling tools can help identify bottlenecks and understand where the time is being spent in your application. Use profiling tools specific to your programming language to gather insights into performance:
- Identify Hotspots: Determine which functions or sections of code consume the most resources and focus your optimization efforts there.
- Run Benchmarks: Implement benchmarks to test the performance of different code snippets. This can guide you in making informed decisions about which optimizations yield the best results.
6. Leverage Parallelism and Concurrency
With the advent of multi-core processors, parallelism and concurrency have become essential for optimizing performance. Utilize threading, multiprocessing, or asynchronous programming to take advantage of modern hardware:
- Threading: For tasks that can run independently, consider using threads to execute multiple operations simultaneously.
- Asynchronous Programming: For I/O-bound operations, leverage asynchronous programming models to keep the application responsive while waiting for resources.
7. Maintain Readability and Maintainability
While optimization is important, it should not come at the cost of code readability and maintainability. Strive to strike a balance between optimization and clarity:
- Comment Your Code: Document your optimizations to ensure that other developers can understand your reasoning.
- Use Meaningful Variable Names: Clear variable names help convey the purpose of the code, making it easier to maintain and optimize in the future.
Conclusion
Code optimization is a critical aspect of developing efficient and fast applications, especially for large projects. By implementing the strategies outlined above—selecting appropriate algorithms, minimizing I/O operations, optimizing loops, reducing function call overhead, profiling, leveraging parallelism, and maintaining readability—you can significantly enhance the performance of your code. Remember that optimization is an ongoing process; continuously monitor and refine your code as your project evolves. By prioritizing performance from the outset, you will create robust applications that stand the test of time.